Envision a website that’s not just sleek and modern but also engages your visitors, leaving a lasting impression. In this article, we’ll explain React, highlight the benefits of integrating it with your WordPress site, and provide a step-by-step guide for setting it up. We’ll also cover essential considerations and show you how to get started with ReactJS to maximize your online presence.
What is React?
ReactJS is an open-source JavaScript library developed by Facebook that makes building user interfaces (UIs) for your website a breeze. It’s different from using a JavaScript framework. A Javascript framework will enable you to do routing and data fetching; however, it is inflexible when it comes to how you structure your code. On the other hand, React is a powerful library that provides tools and features that make it easier to build complex UIs. It’s perfect if you want your website to be highly dynamic and responsive. Moreover, React uses a unique syntax called JSX, that fuses JavaScript and HTML, which makes it easy to read and code with.
Here’s a summary of the main features of React, its benefits, and applications:
Feature | Explanation | Main benefit |
Declarative syntax | Allows developers to describe how the UI should look for different states of the application. | Improves predictability and understanding of UI. |
Component-based architecture | Applications are built using reusable components, each with its own logic and rendering. | Easier to manage and scale applications. |
Virtual DOM | Optimizes updates to the actual DOM by updating a virtual DOM and then applying changes to the real DOM. | Improves performance and efficiency. |
JSX | A syntax extension that allows HTML to be written within JavaScript code. | Easier to understand and debug code. |
Unidirectional data flow | Data flows in a single direction through the application, making managing and predicting state changes easier. | Predictable and manageable state changes. |
Lifecycle methods | Components have methods that are called at different stages of their lifecycle, providing hooks for running code. | Fine-grained control over component behavior. |
Hooks | Allows use of state and other features without writing a class. | Simplifies the use of state and features. |
Context API | Allows to share values across the application without passing props through multiple layers. | Simplifies state management in large applications. |
Server-Side Rendering (SSR) | Supports rendering React components on the server to improve performance and SEO. | Improves performance and SEO. |
React Native | Framework for building mobile applications using React. | Applies React knowledge to mobile development. |
Rich ecosystem and community | Provides access to numerous resources, third-party libraries, and community support. | Access to resources and support. |
What tools do you need to build a web app with React and WordPress?
Creating your website Using React and WordPress requires the help of a few tools to streamline your development process and build a performant, scalable application. More specifically, you’ll need to use tools to set up a local development environment, manage APIs, code efficiently, and ensure version control. Additionally, specific libraries and frameworks tailored for React and WordPress integration will streamline your workflow and enhance the overall development experience.
If you’re looking for the assistance of technical experts in the field, you can submit your project to Codeable and hire an expert to help you with this process. Our skilled WordPress developers are adept in web development with such tools. They’ll even help you with everything from plugin creation or customization to website design and development.
On the other hand, if you are interested in learning more about what it takes to build your website with WP and React, here are the tools you’ll need and why you’ll need them:
For WordPress setup and API management
WordPress, by default, includes the WordPress REST API as a standard feature in all up-to-date versions. This will allow you to integrate React with your WordPress website seamlessly. Moreover, plugins like WP REST API Controller can manage API endpoints through a user interface, making it easier for you to develop your WordPress website using React.
For development environment and version control
Localhost tools, such as XAMPP or MAMP, will enable you to create and test your website on your computer. These tools will help you create a local WordPress environment to develop, test, and debug your application without affecting your live site. Once you are happy with the changes, you can deploy the website to your live server for others to see.
Visual Studio Code or Atom are powerful text editors that make writing and editing code more efficient. They provide syntax highlighting, autocompletion, and extension support, which are useful features you’ll need when coding with React. Additionally, a version control like GitHub will keep your code and its versions organized.
React development tools
The JavaScript runtime environment, Node.js, is an essential tool for modern web development and streamlining the React development process. Additionally, the npm and create-react-app packages handle the initial configuration and setup of the development environment. They bundle all the necessary dependencies and create a basic project structure for your React app.
The Axios library facilitates making HTTP requests from your React app to your WordPress website. This is particularly useful if you’re using the WP REST API.
React frameworks and boilerplates
These play a crucial role in developing your React app because they will provide ready-to-use tools, standards, and templates to facilitate your development process. Some popular React frameworks include Frontity, Faust, Gatsby, and Next. Gatsby and Next.js are React frameworks designed explicitly for generating static sites and rendering content on your server. On the other hand, Frontity and Faust are designed to work with WordPress and have pre-built components and libraries that can help you create your modern and responsive headless CMS site quickly and easily.
You might need any of these tools while building your application with React and WordPress.
Setting up WordPress React (Headless WordPress with React as the front-end)
This step-by-step guide will walk you through setting up a React project that renders WordPress post data:
Step 1: How to create a new project with React
- Install Node.js and npm: Ensure you have Node.js and npm installed on your computer.
- Install required packages: You’ll need the create-react-app package to set up a new React project. If you don’t have it installed, you can install it globally using npm:
npm install -g create-react-app
You might also need Axios:
npm install axios
import axios from 'axios';
- Create a new React project: Open your terminal and run the following command to create a new React project. Make sure to replace my-react-app with your preferred project name:
npx create-react-app my-react-app
- Navigate to your project directory by running the following command:
cd my-react-app
- Start the development server: Run the following command to start your React development server:
npm start
Your new React app should now be running on http://localhost:3000.
Step 2: How to render post data on React
- Set up WordPress REST API: Ensure your WordPress site has the REST API enabled by logging in to your WordPress site’s admin panel and navigating to Settings > Permalinks. Ensure that your permalinks are enabled and set to something other than Plain. This is a requirement for the REST API to function properly.
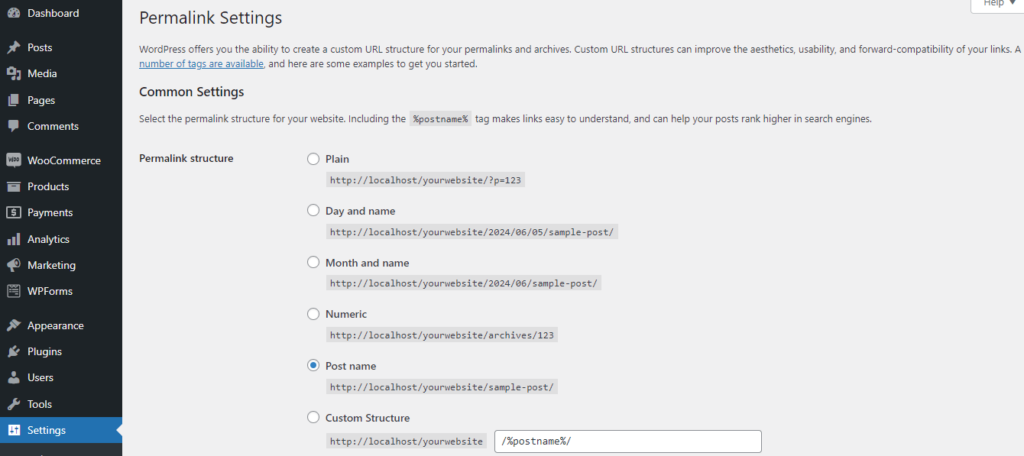
Then, go to Settings> Reading. Scroll down to the bottom of the Reading Settings page. You should see an option labeled Discourage search engines from indexing this site. Ensure that this option is unchecked. If it is checked, it can prevent the REST API from working as expected.
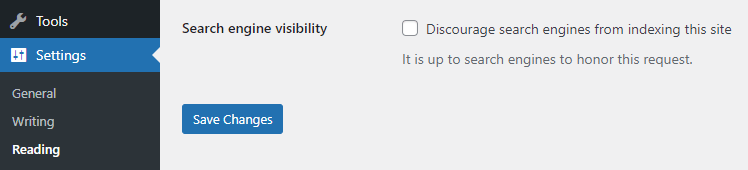
- Confirm that the REST API is enabled: To check if the REST API is enabled and working properly, access it directly in your browser. Open a new browser tab and enter the following URL:
http://your-wordpress-site/wp-json/wp/v2/posts
Replace your-wordpress-site with the URL of your WordPress site. If the REST API is enabled, you should see a JSON response containing information about your WordPress posts.
- Install and activate the WPGraphQL plugin: Go to your WordPress admin panel > Plugins > Add New Plugin. Search for WPGraphQL, click Install Now, then Activate.
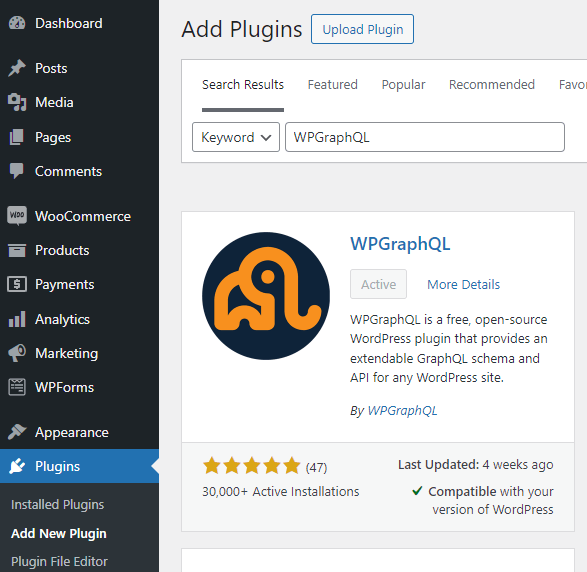
- Create an Apollo Client instance to interact with your GraphQL server.
// src/apolloClient.js
import { ApolloClient, InMemoryCache, HttpLink } from "@apollo/client";
const client = new ApolloClient({
link: new HttpLink({
uri: "http://localhost/yourwebsite/graphql",
}),
cache: new InMemoryCache(),
});
export default client;
- Set a component to fetch your WordPress posts:
// src/components/PostList.js import React from “react”; import { useQuery } from “@apollo/client”; import { GET_POSTS } from “../graphql/queries”; const PostList = () => { const { loading, error, data } = useQuery(GET_POSTS); if (loading) return <p>Loading…</p>; if (error) return <p>Error :(</p>; return ( <div> {data.posts.nodes.map(({ id, title, content }) => ( <div key={id}> <h2>{title}</h2> <p>{content}</p> </div> ))} </div> ); }; export default PostList; // src/graphql/queries.js import { gql } from “@apollo/client”; export const GET_POSTS = gql` query GetPosts { posts { nodes { id title content } } } } `; |
Open the src/App.js file in your project. Replace the content with the following code:
import logo from "./logo.svg";
import "./App.css";
// src/App.js
import React from "react";
import { ApolloProvider } from "@apollo/client";
import client from "./apolloClient";
import PostList from "./components/PosttList";
const App = () => {
return (
<ApolloProvider client={client}>
<div>
<h1>Headless WooCommerce Store</h1>
<PostList />
</div>
</ApolloProvider>
);
};
export default App;
Step 3: Styling the posts
Now it’s time to add some basic styling to your posts. You can do this in two ways:
- Manually, using CSS by creating a PostList.css file and importing it in PostList.js.
- Using ready-made style libraries and frameworks like React Bootstrap, Material-UI, Semantic UI React, or Tailwind CSS.
Two ways you can use React with WordPress
There are several advanced ways you can integrate React into a WordPress website. Choosing from the following top approaches will depend on your goals and preferences for your WordPress website.
Creating a custom WordPress theme using React
Creating a custom WordPress theme using React can be highly beneficial for developing modern, interactive, and performant websites. This approach is particularly advantageous for projects where a high degree of interactivity is needed.
Create React WPTheme is a tool that helps developers create modern WordPress themes using the React JavaScript library. It provides a boilerplate and development environment for building React-based WordPress themes.
It is similar to Create React App, which we used earlier, but it uses WordPress instead of Webpack as the development server. This allows consolidating front-end and back-end development in one place. It gives access to WordPress core functions, hooks, actions, filters, etc., within the React theme. You can also still use plain PHP and WordPress page templates if needed.
The react-src directory will contain the uncompiled, editable React code. Everything else, including the root and static folders, are outputs of what’s in react-src.
To create a new theme:
- Open your terminal and navigate to the wp-content/themes directory of your WordPress installation.
- Clone the Create React WPTheme repository:
git clone https://github.com/devloco/create-react-wptheme.git my-custom-theme
- Navigate to your theme directory:
cd my-custom-theme
- Open theme.json in your theme’s root directory and Customize the metadata (name, description, author, etc.) according to your needs.
- Create your React components in the src directory. Use standard React practices and structure to develop your components as we did previously.
- Finally, from your WordPress dashboard, go to Appearance > Themes, then activate your theme.
Create a custom Gutenberg block using React
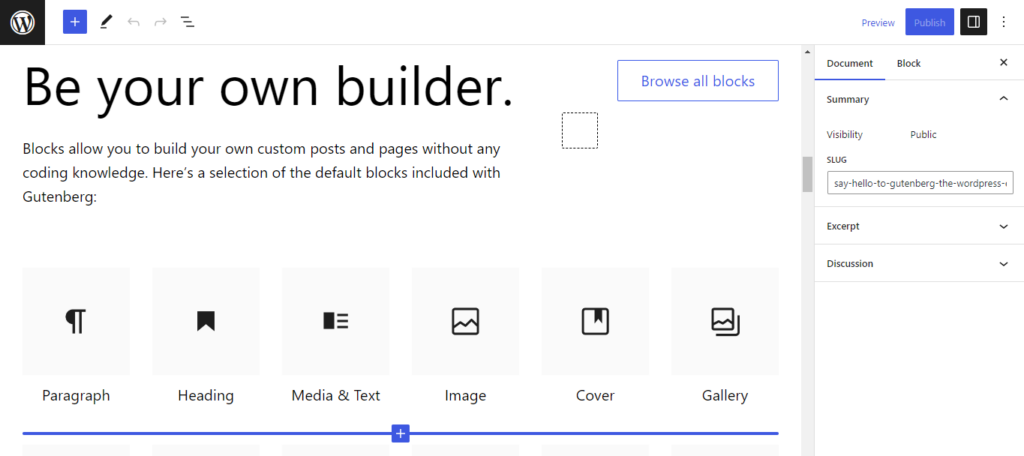
Creating custom Gutenberg blocks using React is a powerful way to extend the WordPress block editor’s capabilities. It allows developers to provide tailored, reusable, and interactive content elements that enhance the user experience and maintain consistency across a site.
Leveraging React’s strengths, developers can efficiently build and manage complex UI components, ensuring that their WordPress sites can meet specific requirements and deliver high-quality content presentation. Here’s how:
- Create a new WordPress plugin or theme folder to house your custom block code.
- Initialize a new npm project inside the folder.
- Install the @wordpress/scripts package, which provides helpful scripts for building blocks.
- Create a PHP file (e.g., my-custom-block.php) to register your block with WordPress
- Use the register_block_type function and point it to the compiled JS file for your block.
- Set up your block’s React component. Create a src folder and add your main block JS file, e.g., index.js.
- Create your block’s edit and save functions that return JSX.
- Register the block in the registerBlockType function then compile your block’s JavaScript.
- Add a build script to your package.json that uses wp-scripts.
- Run npm run build to compile your block’s JS to the build folder.
- Enqueue your block’s assets
- Create a block.json file to define your block’s metadata
- Specify the path to the compiled index.js file like so, for example:
{
"apiVersion": 2,
"name": "custom-namespace/custom-block-name",
"title": "Custom Block",
"category": "widgets",
"icon": "exampleicon",
"editorScript": "file:./build/index.js"
}
That covers the basic setup! You can now activate your plugin/theme, and your custom React-powered block should appear in the block inserter in the WordPress editor.
On the other hand, if you are interested in learning more about what it takes to build your website with WP and React, here are the tools you’ll need and why you’ll need them:
- WordPress Install, WordPress REST API, and WP REST API Controller
WordPress, by default, includes the WordPress REST API as a standard feature in all up-to-date versions. This will allow you to integrate React with your WordPress website seamlessly. Moreover, plugins like WP REST API Controller can manage API endpoints through a user interface. This will make it easier for your to develop your WordPress website using React.
- Local Development Environment (LDE)
Localhost tools, such as XAMPP or MAMP, will enable you to create and test your website on your computer. These tools will help you create a local WordPress environment to develop, test, and debug your application without affecting your live site. Once you are happy with the changes, you can deploy the website to your live server for others to see.
- Code Editor and Version Control
Visual Studio Code or Atom are powerful text editors that make writing and editing code more efficient. They provide syntax highlighting, autocompletion, and extension support, which are useful features you’ll need when coding with React. Additionally, a version control like GitHub will keep your code and its versions organized.
- Node.js, npm, and the create-react-app
The JavaScript runtime environment, Node.js, is an essential tool for modern web development and streamlining the React development process. Additionally, the npm and create-react-app packages handle the initial configuration and setup of the development environment. They bundle all the necessary dependencies and create a basic project structure for your React app.
- The Axios Library
The Axios library facilitates making HTTP requests from your React app to your WordPress website. This is particularly useful if you’re using the WP REST API.
- React Frameworks and Boilerplates
These play a crucial role in developing your React app because they will provide you with ready-to-use tools, standards, and templates that will facilitate your development process. Some popular React frameworks include Frontity, Faust, Gatsby, and Next. Furthermore, Gatsby and Next.js are React frameworks specifically designed for generating static sites and rendering content on your server side. On the other hand, Frontity and Faust are designed to work with WordPress and have pre-built components and libraries that can help you create your modern and responsive headless CMS site quickly and easily.
Key considerations when building your web app with React and WP
When considering integrating React into a WordPress site, it’s important to choose the approach that will be efficient for your website needs based on the following aspects:
Existing infrastructure
The infrastructure you have in place can affect compatibility, scalability, development time, and cost. Choosing an approach that is compatible with your existing infrastructure can help ensure a smoother and more efficient development process. For example, a headless setup may be more efficient for your website needs. However, it requires, more development effort, back-end changes, expertise, and resources.
Maintenance and updates
Regular maintenance and updates are crucial when developing a website using React with WordPress. They benefit your website’s performance, security, and stability. Compatibility and security vulnerabilities may arise without regular updates, resulting in a poor user experience and potential site crashes. The headless setup simplifies updates, as front-end and back-end updates are separate.
Developer skillset
Building your website with WordPress and React is a time-consuming process with a steep learning curve. Therefore, having a sufficient developer skillset is crucial to working with JavaScript, JSX, and the tools mentioned earlier. A proficient React developer should have strong knowledge of JavaScript ES6+, an understanding of JSX syntax, experience with state management libraries like Redux or Context API, and familiarity with modern front-end build tools like Webpack and Babel. Additionally, expertise in RESTful APIs and asynchronous programming (e.g., Promises, async/await) is essential.
To have easy access to these skills, hiring an expert on a project basis via Codeable can seamlessly facilitate this process.
SEO considerations
SEO is important for your website’s discoverability and ranking in search results, which can increase your conversion rates and profit. When using React with WordPress, it’s important to consider how it will affect SEO, such as how search engines will crawl and index your content. React’s impact on SEO primarily revolves around its handling of dynamic content. Since React uses client-side rendering by default, it can cause issues for search engines that struggle to index JavaScript-heavy content. Implementing server-side rendering (SSR) with frameworks like Next.js or static site generation (SSG) with Gatsby can significantly improve SEO. Ensuring that metadata, sitemaps, and canonical URLs are properly set up in a headless WordPress setup is also crucial for effective SEO. Moreover, a headless setup may require extra effort to implement SEO best practices for effective search engine optimization.
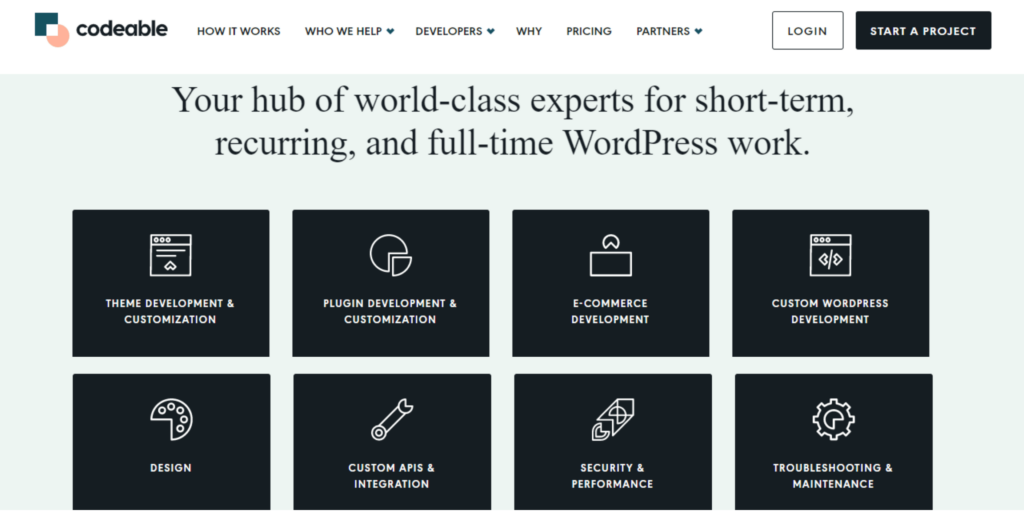
Best practices when configuring react
Configuring React to work with your WordPress requires careful attention to several key aspects. This section of code improves code organization, maintainability, reusability, scalability, and testability and tells you about the best practices you’ll need to implement in your project. These best practices are provided by Codeable expert Robert Meszaros, whose areas of specialization include site migration and custom API integration.
Write modular code
By breaking your code into smaller, self-contained modules, you can manage and reuse it more easily, ensuring that it works as expected
Therefore, it’s best to write reusable, modular React components to keep your codebase organized and maintainable.
Use a state management library
Using a state management library provides a centralized way of managing the state of your application, making it easier to build and maintain larger and more complex applications. Additionally, it can simplify managing and updating your website while providing modularity and testability benefits. If you’re developing a complex website, consider using a state management library like Redux to manage the application efficiently and predictably.
Optimize performance
Optimizing performance improves the user experience by reducing load time nd response time. To optimize performance, you should consider features such as caching static assets, code splitting, and lazy loading. These best practices will improve the site’s speed and user experience.
Implement a consistent API
A consistent and well-documented API like WordPress REST API or GraphQL simplifies the data fetching between your React app and WordPress website. It ensures seamless integration and reliable performance. When choosing the API you’ll work with, consider that there are certain principles and constraints that define RESTful architecture. No official standards or specifications define how a RESTful API should be designed or implemented. GraphQL APIs, on the other hand, tend to follow a more consistent and predictable pattern. The functions of a GraphQL API will depend on how it is designed and implemented by its developer. This is an excellent guide that compares GraphQL APIs with RESTful APIs.
Plan for extensibility
Designing your website’s architecture with extensibility in mind allows for more flexibility, scalability, modularization, and reusability in the long run. It future-proofs your website and enables you to easily add new features or changes without affecting your existing codebase.
Automated testing
Automated testing saves time and ensures consistency, scalability, reliability, and stability. It helps you identify errors early on and prevents issues from frustrating your website’s visitors.
Hiring Robert Meszaros will be a smart choice if you want to build your website using WordPress and React through a skilled developer who will follow best practices He has extensive experience in clean code architecture, version control, and testing. He’s also dedicated to staying up-to-date with the ever-evolving industry advancements.
Build your website using WP and React with the Help of Codeable
Using React alongside WordPress is a powerful way to create a flexible, scalable, and highly performant website. With WordPress as the CMS backbone, you get the benefits of its content management system while still having the ability to use React to create custom components or an entirely decoupled front-end. However, this process can be complicated and challenging if you don’t have sufficient coding experience. Fortunately, our Codeable experts can help you overcome this obstacle and can even be filtered by their experience in React development. With our library of experts, you can be confident that you’ll find a developer to meet your exact technical needs. Whether you want to create a new web app or optimize your existing website, just submit your project to Codeable and let our experts carry out all your technical needs.